Stay updated with job positions, news and more INSCALE
Featured articles
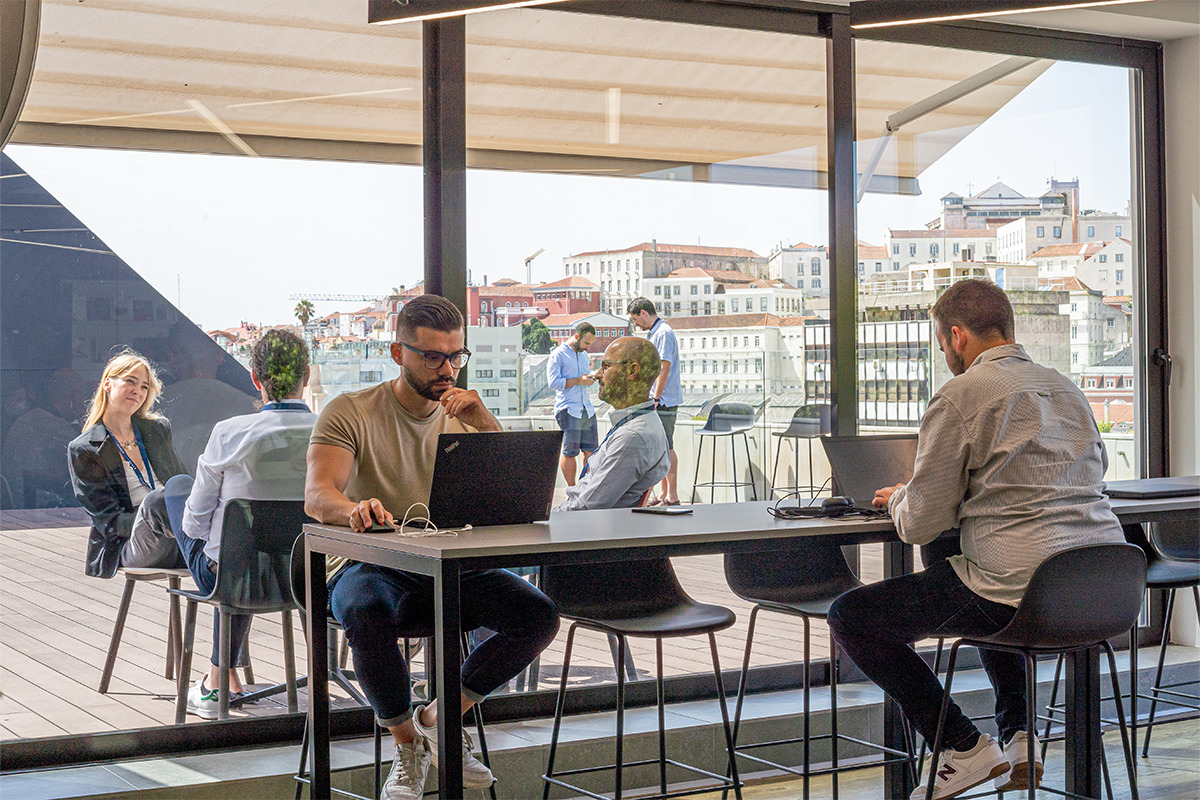
Benefits of Working with an IT Recruitment Specialist Instead of Hiring on Your Own
In today’s fast-paced business world, finding the right talent for your IT department can be a challenging task. Many organisations are faced with the question
Benefits of Building a Tech Hub Abroad
In today’s world, businesses are constantly seeking new ways to stay competitive and innovative. One of the strategies that has been stable for many years,
The Key to a Successful Recruitment Process —11 Steps to Help You Hire the Right Candidate
The right person in the right position can truly work wonders for any company. However, it requires knowing how, and where to search, to find
Everything You Need to Know About Talent Acquisition
Talent acquisition is a term that is becoming increasingly common when talking about recruitment. It’s not surprising since a good talent acquisition strategy can create
What Does IT Recruitment Really Cost?
What does recruitment actually cost? The short answer is that recruitment can come with a price tag ranging from a few thousand up to 80.000
Why do INSCALE only work with Talent Acquisition of Tech Talents?
INSCALE is not your average recruitment agency. Instead of being a generalist agency, we have a niche focus on the tech industry, and we pride
Why consider Macedonia as a tech-hub for your development
In today’s interconnected world, harnessing the power of global tech talent has become a cornerstone of success for businesses of all sizes. One location that’s
Embrace Shared Hiring Risk: The Game-Changer for Employers
Hiring new personnel is always a lengthy and often risky process. Will the new candidate be a good fit, will they stay with the company,
How to Effectively Hire a Software Engineer
Hiring a software engineer or developer can be a daunting and challenging task, but with the right approach and preparation, it can be both smooth
Mistakes to Avoid in Tech Recruitment
Hiring top-notch tech talent is the cornerstone of building a successful and innovative organisation in today’s fast-paced digital landscape. However, the journey to find the
Hire Now!
Are you looking to expand your team with exceptional talent? Now is the perfect time to act! As the summer holidays approach, job searches and
Tech Talent is Crucial for PropTech, but It’s Becoming Challenging to Find in Sweden
Competitive Advantage In a constantly evolving market, securing top tech talent provides a competitive edge to property tech companies. Skilled professionals play a vital role
World PassWord Day
World Password Day is an annual event that occurs on the first Thursday of every May to encourage people to adopt strong password habits and
ISO 27001 Compliance!
Our office in Lisbon has been awarded the ISO/IEC 27001 certification for our information security management system (ISMS). Last year we received the ISO 27001
Why INSCALE is the Right Partner for Your Business
In 2023, the International Fintech Summit (IFGS) will bring together a diverse range of industry leaders for two days of thought-provoking discussions. Attendees will include
A Guide to Successful Tech Talent Recruitment
Technology is playing a significant role in the world of business today and having a strong team of tech experts is crucial to success. However,
World BackUp Day 2023
Today, many may not consider the importance of backup, as cloud solutions have become ubiquitous, and data is automatically saved. However, does this imply that
5 Proven Strategies for Keeping Your Developers Productive and Happy
In today’s fast-paced digital world, software development is a critical component of many organizations. As such, it is essential to keep developers productive and happy
Talent as a Service – The Critical Transition to TaaS 2023
Are you interested in a more efficient and effective way to find top-tier talent for your company? Then you might want to learn about Talent
ISO 27001 Compliance!
Being an important wheel in the ecosystem of software engineering and wanting to be in the frontline with our clients, INSCALE choose to be audited
INSCALE joins HackerX – where developers meet their new opportunities
We are very excited to have two of our representatives partaking in the HackerX Lisbon event on the 11 of May 2023. HackerX is the